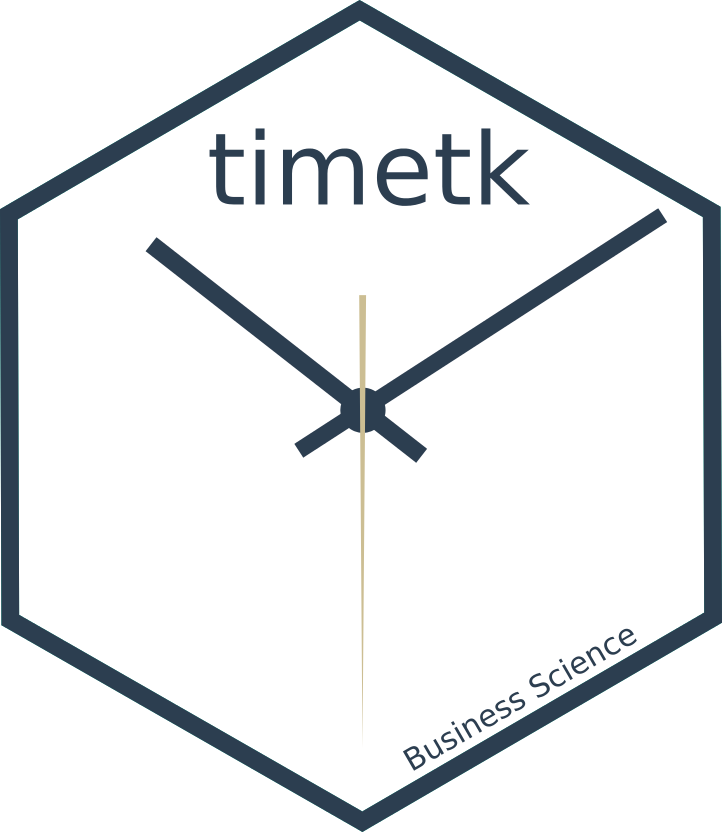
Coerce time series objects and tibbles with date/date-time columns to ts.
Source:R/coersion-tk_zooreg.R
tk_zooreg.Rd
Coerce time series objects and tibbles with date/date-time columns to ts.
Usage
tk_zooreg(
data,
select = NULL,
date_var = NULL,
start = 1,
end = numeric(),
frequency = 1,
deltat = 1,
ts.eps = getOption("ts.eps"),
order.by = NULL,
silent = FALSE
)
tk_zooreg_(
data,
select = NULL,
date_var = NULL,
start = 1,
end = numeric(),
frequency = 1,
deltat = 1,
ts.eps = getOption("ts.eps"),
order.by = NULL,
silent = FALSE
)
Arguments
- data
A time-based tibble or time-series object.
- select
Applicable to tibbles and data frames only. The column or set of columns to be coerced to
zooreg
class.- date_var
Applicable to tibbles and data frames only. Column name to be used to
order.by
.NULL
by default. IfNULL
, function will find the date or date-time column.- start
the time of the first observation. Either a single number or a vector of two integers, which specify a natural time unit and a (1-based) number of samples into the time unit.
- end
the time of the last observation, specified in the same way as
start
.- frequency
the number of observations per unit of time.
- deltat
the fraction of the sampling period between successive observations; e.g., 1/12 for monthly data. Only one of
frequency
ordeltat
should be provided.- ts.eps
time series comparison tolerance. Frequencies are considered equal if their absolute difference is less than
ts.eps
.- order.by
a vector by which the observations in
x
are ordered. If this is specified the argumentsstart
andend
are ignored andzoo(data, order.by, frequency)
is called. Seezoo
for more information.- silent
Used to toggle printing of messages and warnings.
Details
tk_zooreg()
is a wrapper for zoo::zooreg()
that is designed
to coerce tibble
objects that have a "time-base" (meaning the values vary with time)
to zooreg
class objects. There are two main advantages:
Non-numeric columns get removed instead causing coercion issues.
If an index is present, the returned
zooreg
object retains an index retrievable usingtk_index()
.
The select
argument is used to select subsets
of columns from the incoming data.frame.
The date_var
can be used to specify the column with the date index.
If date_var = NULL
, the date / date-time column is interpreted.
Optionally, the order.by
argument from the underlying xts::xts()
function can be used.
The user must pass a vector of dates or date-times if order.by
is used.
Only columns containing numeric data are coerced.
At a minimum, a frequency
and a start
should be specified.
For non-data.frame object classes (e.g. xts
, zoo
, timeSeries
, etc) the objects are coerced
using zoo::zooreg()
.
tk_zooreg_
is a nonstandard evaluation method.
Examples
### tibble to zooreg: Comparison between tk_zooreg() and zoo::zooreg()
data_tbl <- tibble::tibble(
date = seq.Date(as.Date("2016-01-01"), by = 1, length.out = 5),
x = rep("chr values", 5),
y = cumsum(1:5),
z = cumsum(11:15) * rnorm(1))
# zoo::zooreg: Values coerced to character; Result does not retain index
data_zooreg <- zoo::zooreg(data_tbl[,-1], start = 2016, freq = 365)
data_zooreg # Numeric values coerced to character
#> x y z
#> 2016(1) chr values 1 -4.263393
#> 2016(2) chr values 3 -8.914366
#> 2016(3) chr values 6 -13.952921
#> 2016(4) chr values 10 -19.379057
#> 2016(5) chr values 15 -25.192774
rownames(data_zooreg) # NULL, no dates retained
#> NULL
# tk_zooreg: Only numeric columns get coerced; Result retains index as rownames
data_tk_zooreg <- tk_zooreg(data_tbl, start = 2016, freq = 365)
#> Warning: Non-numeric columns being dropped: date, x
data_tk_zooreg # No inadvertent coercion to character class
#> y z
#> 2016(1) 1 -4.263393
#> 2016(2) 3 -8.914366
#> 2016(3) 6 -13.952921
#> 2016(4) 10 -19.379057
#> 2016(5) 15 -25.192774
# timetk index
tk_index(data_tk_zooreg, timetk_idx = FALSE) # Regularized index returned
#> [1] 2016.000 2016.003 2016.005 2016.008 2016.011
tk_index(data_tk_zooreg, timetk_idx = TRUE) # Original date index returned
#> [1] "2016-01-01" "2016-01-02" "2016-01-03" "2016-01-04" "2016-01-05"
### Using select and date_var
tk_zooreg(data_tbl, select = y, date_var = date, start = 2016, freq = 365)
#> y
#> 2016(1) 1
#> 2016(2) 3
#> 2016(3) 6
#> 2016(4) 10
#> 2016(5) 15
### NSE: Enables programming
select <- "y"
date_var <- "date"
tk_zooreg_(data_tbl, select = select, date_var = date_var, start = 2016, freq = 365)
#> y
#> 2016(1) 1
#> 2016(2) 3
#> 2016(3) 6
#> 2016(4) 10
#> 2016(5) 15