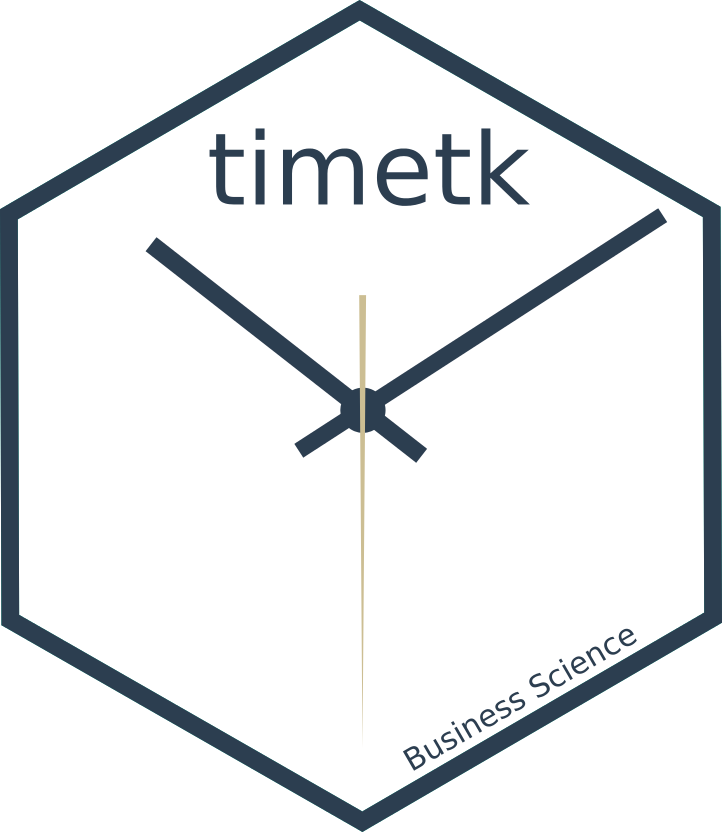
Coerce time series objects and tibbles with date/date-time columns to ts.
Source:R/coersion-tk_ts.R
tk_ts.Rd
Coerce time series objects and tibbles with date/date-time columns to ts.
Arguments
- data
A time-based tibble or time-series object.
- select
Applicable to tibbles and data frames only. The column or set of columns to be coerced to
ts
class.- start
the time of the first observation. Either a single number or a vector of two numbers (the second of which is an integer), which specify a natural time unit and a (1-based) number of samples into the time unit. See the examples for the use of the second form.
- end
the time of the last observation, specified in the same way as
start
.- frequency
the number of observations per unit of time.
- deltat
the fraction of the sampling period between successive observations; e.g., 1/12 for monthly data. Only one of
frequency
ordeltat
should be provided.- ts.eps
time series comparison tolerance. Frequencies are considered equal if their absolute difference is less than
ts.eps
.- silent
Used to toggle printing of messages and warnings.
Details
tk_ts()
is a wrapper for stats::ts()
that is designed
to coerce tibble
objects that have a "time-base" (meaning the values vary with time)
to ts
class objects. There are two main advantages:
Non-numeric columns get removed instead of being populated by NA's.
The returned
ts
object retains a "timetk index" (and various other attributes) if detected. The "timetk index" can be used to coerce betweentbl
,xts
,zoo
, andts
data types.
The select
argument is used to select subsets
of columns from the incoming data.frame.
Only columns containing numeric data are coerced. At a minimum, a frequency
and a start
should be specified.
For non-data.frame object classes (e.g. xts
, zoo
, timeSeries
, etc) the objects are coerced
using stats::ts()
.
tk_ts_
is a nonstandard evaluation method.
Examples
library(dplyr)
### tibble to ts: Comparison between tk_ts() and stats::ts()
data_tbl <- tibble::tibble(
date = seq.Date(as.Date("2016-01-01"), by = 1, length.out = 5),
x = rep("chr values", 5),
y = cumsum(1:5),
z = cumsum(11:15) * rnorm(1))
# as.ts: Character columns introduce NA's; Result does not retain index
stats::ts(data_tbl[,-1], start = 2016)
#> Time Series:
#> Start = 2016
#> End = 2020
#> Frequency = 1
#> x y z
#> 2016 1 1 -8.991532
#> 2017 1 3 -18.800476
#> 2018 1 6 -29.426831
#> 2019 1 10 -40.870599
#> 2020 1 15 -53.131779
# tk_ts: Only numeric columns get coerced; Result retains index in numeric format
data_ts <- tk_ts(data_tbl, start = 2016)
#> Warning: Non-numeric columns being dropped: date, x
data_ts
#> Time Series:
#> Start = 2016
#> End = 2020
#> Frequency = 1
#> y z
#> 2016 1 -8.991532
#> 2017 3 -18.800476
#> 2018 6 -29.426831
#> 2019 10 -40.870599
#> 2020 15 -53.131779
#> attr(,"index")
#> [1] 1451606400 1451692800 1451779200 1451865600 1451952000
#> attr(,"index")attr(,"tzone")
#> [1] UTC
#> attr(,"index")attr(,"tclass")
#> [1] Date
# timetk index
tk_index(data_ts, timetk_idx = FALSE) # Regularized index returned
#> [1] 2016 2017 2018 2019 2020
tk_index(data_ts, timetk_idx = TRUE) # Original date index returned
#> Warning: 'tzone' attributes are inconsistent
#> [1] "2016-01-01" "2016-01-02" "2016-01-03" "2016-01-04" "2016-01-05"
# Coerce back to tibble
data_ts %>% tk_tbl(timetk_idx = TRUE)
#> Warning: 'tzone' attributes are inconsistent
#> # A tibble: 5 × 3
#> index y z
#> <date> <dbl> <dbl>
#> 1 2016-01-01 1 -8.99
#> 2 2016-01-02 3 -18.8
#> 3 2016-01-03 6 -29.4
#> 4 2016-01-04 10 -40.9
#> 5 2016-01-05 15 -53.1
### Using select
tk_ts(data_tbl, select = y)
#> Time Series:
#> Start = 1
#> End = 5
#> Frequency = 1
#> y
#> [1,] 1
#> [2,] 3
#> [3,] 6
#> [4,] 10
#> [5,] 15
#> attr(,"index")
#> [1] 1451606400 1451692800 1451779200 1451865600 1451952000
#> attr(,"index")attr(,"tzone")
#> [1] UTC
#> attr(,"index")attr(,"tclass")
#> [1] Date
### NSE: Enables programming
select <- "y"
tk_ts_(data_tbl, select = select)
#> Time Series:
#> Start = 1
#> End = 5
#> Frequency = 1
#> y
#> [1,] 1
#> [2,] 3
#> [3,] 6
#> [4,] 10
#> [5,] 15
#> attr(,"index")
#> [1] 1451606400 1451692800 1451779200 1451865600 1451952000
#> attr(,"index")attr(,"tzone")
#> [1] UTC
#> attr(,"index")attr(,"tclass")
#> [1] Date