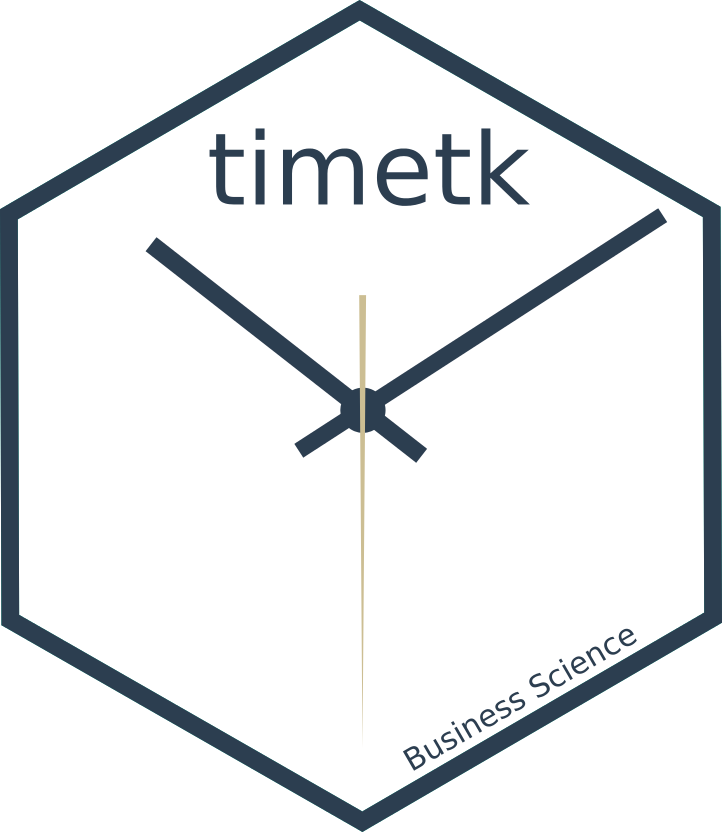
Extract an index of date or datetime from time series objects, models, forecasts
Source:R/index-tk_index.R
tk_index.Rd
Extract an index of date or datetime from time series objects, models, forecasts
Arguments
- data
A time-based tibble, time-series object, time-series model, or
forecast
object.- timetk_idx
If
timetk_idx
isTRUE
a timetk time-based index attribute is attempted to be returned. IfFALSE
the default index is returned. See discussion below for further details.- silent
Used to toggle printing of messages and warnings.
Details
tk_index()
is used to extract the date or datetime index from various
time series objects, models and forecasts.
The method can be used on tbl
, xts
, zoo
, zooreg
, and ts
objects.
The method can additionally be used on forecast
objects and a number of
objects generated by modeling functions such as Arima
, ets
, and HoltWinters
classes to get the index of the underlying data.
The boolean timetk_idx
argument is applicable to regularized time series objects
such as ts
and zooreg
classes that have both a regularized index and potentially
a "timetk index" (a time-based attribute).
When set to FALSE
the regularized index is returned.
When set to TRUE
the time-based timetk index is returned if present.
has_timetk_idx()
is used to determine if the object has a "timetk index" attribute
and can thus benefit from the tk_index(timetk_idx = TRUE)
.
TRUE
indicates the "timetk index" attribute is present.
FALSE
indicates the "timetk index" attribute is not present.
If FALSE
, the tk_index()
function will return the default index for the data type.
Important Note: To gain the benefit of timetk_idx
the time series
must have a timetk index.
Use has_timetk_idx
to determine if the object has a timetk index.
This is particularly important for ts
objects, which
by default do not contain a time-based index and therefore must be coerced from time-based
objects such as tbl
, xts
, or zoo
using the tk_ts()
function in order
to get the "timetk index" attribute.
Refer to tk_ts()
for creating persistent date / datetime index
during coercion to ts
.
Examples
# Create time-based tibble
data_tbl <- tibble::tibble(
date = seq.Date(from = as.Date("2000-01-01"), by = 1, length.out = 5),
x = rnorm(5) * 10,
y = 5:1
)
tk_index(data_tbl) # Returns time-based index vector
#> [1] "2000-01-01" "2000-01-02" "2000-01-03" "2000-01-04" "2000-01-05"
# Coerce to ts using tk_ts(): Preserves time-basis
data_ts <- tk_ts(data_tbl)
#> Warning: Non-numeric columns being dropped: date
tk_index(data_ts, timetk_idx = FALSE) # Returns regularized index
#> [1] 1 2 3 4 5
tk_index(data_ts, timetk_idx = TRUE) # Returns original time-based index vector
#> Warning: 'tzone' attributes are inconsistent
#> [1] "2000-01-01" "2000-01-02" "2000-01-03" "2000-01-04" "2000-01-05"
# Coercing back to tbl
tk_tbl(data_ts, timetk_idx = FALSE) # Returns regularized tbl
#> Warning: Warning: No index to preserve. Object otherwise converted to tibble successfully.
#> # A tibble: 5 × 2
#> x y
#> <dbl> <dbl>
#> 1 -23.9 5
#> 2 7.82 4
#> 3 -10.0 3
#> 4 12.9 2
#> 5 -5.35 1
tk_tbl(data_ts, timetk_idx = TRUE) # Returns time-based tbl
#> Warning: 'tzone' attributes are inconsistent
#> # A tibble: 5 × 3
#> index x y
#> <date> <dbl> <dbl>
#> 1 2000-01-01 -23.9 5
#> 2 2000-01-02 7.82 4
#> 3 2000-01-03 -10.0 3
#> 4 2000-01-04 12.9 2
#> 5 2000-01-05 -5.35 1