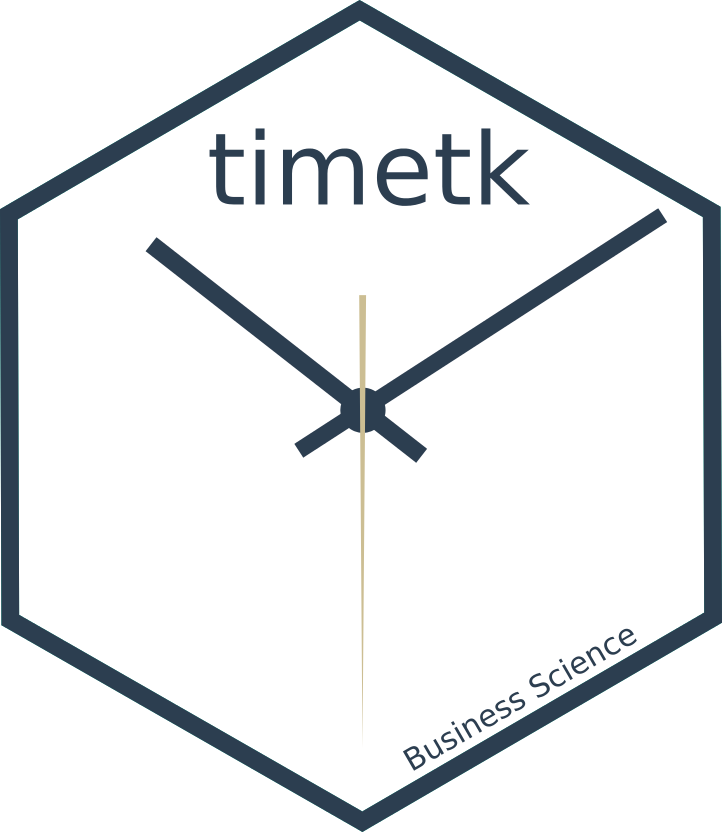
Make daily Holiday and Weekend date sequences
Source:R/make-tk_make_holiday_sequences.R
tk_make_holiday_sequence.Rd
Make daily Holiday and Weekend date sequences
Usage
tk_make_holiday_sequence(
start_date,
end_date,
calendar = c("NYSE", "LONDON", "NERC", "TSX", "ZURICH"),
skip_values = NULL,
insert_values = NULL
)
tk_make_weekend_sequence(start_date, end_date)
tk_make_weekday_sequence(
start_date,
end_date,
remove_weekends = TRUE,
remove_holidays = FALSE,
calendar = c("NYSE", "LONDON", "NERC", "TSX", "ZURICH"),
skip_values = NULL,
insert_values = NULL
)
Arguments
- start_date
Used to define the starting date for date sequence generation. Provide in "YYYY-MM-DD" format.
- end_date
Used to define the ending date for date sequence generation. Provide in "YYYY-MM-DD" format.
- calendar
The calendar to be used in Date Sequence calculations for Holidays from the
timeDate
package. Acceptable values are:"NYSE"
,"LONDON"
,"NERC"
,"TSX"
,"ZURICH"
.- skip_values
A daily date sequence to skip
- insert_values
A daily date sequence to insert
- remove_weekends
A logical value indicating whether or not to remove weekends (Saturday and Sunday) from the date sequence
- remove_holidays
A logical value indicating whether or not to remove common holidays from the date sequence
Details
Start and End Date Specification
Accept shorthand notation (i.e.
tk_make_timeseries()
specifications apply)Only available in Daily Periods.
Holiday Sequences
tk_make_holiday_sequence()
is a wrapper for various holiday calendars from the timeDate
package,
making it easy to generate holiday sequences for common business calendars:
New York Stock Exchange:
calendar = "NYSE"
Londo Stock Exchange:
"LONDON"
North American Reliability Council:
"NERC"
Toronto Stock Exchange:
"TSX"
Zurich Stock Exchange:
"ZURICH"
Weekend and Weekday Sequences
These simply populate
See also
Intelligent date or date-time sequence creation:
tk_make_timeseries()
Holidays and weekends:
tk_make_holiday_sequence()
,tk_make_weekend_sequence()
,tk_make_weekday_sequence()
Make future index from existing:
tk_make_future_timeseries()
Examples
library(dplyr)
# Set max.print to 50
options_old <- options()$max.print
options(max.print = 50)
# ---- HOLIDAYS & WEEKENDS ----
# Business Holiday Sequence
tk_make_holiday_sequence("2017-01-01", "2017-12-31", calendar = "NYSE")
#> [1] "2017-01-02" "2017-01-16" "2017-02-20" "2017-04-14" "2017-05-29"
#> [6] "2017-07-04" "2017-09-04" "2017-11-23" "2017-12-25"
tk_make_holiday_sequence("2017", calendar = "NYSE") # Same thing as above (just shorter)
#> [1] "2017-01-02" "2017-01-16" "2017-02-20" "2017-04-14" "2017-05-29"
#> [6] "2017-07-04" "2017-09-04" "2017-11-23" "2017-12-25"
# Weekday Sequence
tk_make_weekday_sequence("2017", "2018", remove_holidays = TRUE)
#> [1] "2017-01-03" "2017-01-04" "2017-01-05" "2017-01-06" "2017-01-09"
#> [6] "2017-01-10" "2017-01-11" "2017-01-12" "2017-01-13" "2017-01-17"
#> [11] "2017-01-18" "2017-01-19" "2017-01-20" "2017-01-23" "2017-01-24"
#> [16] "2017-01-25" "2017-01-26" "2017-01-27" "2017-01-30" "2017-01-31"
#> [21] "2017-02-01" "2017-02-02" "2017-02-03" "2017-02-06" "2017-02-07"
#> [26] "2017-02-08" "2017-02-09" "2017-02-10" "2017-02-13" "2017-02-14"
#> [31] "2017-02-15" "2017-02-16" "2017-02-17" "2017-02-21" "2017-02-22"
#> [36] "2017-02-23" "2017-02-24" "2017-02-27" "2017-02-28" "2017-03-01"
#> [41] "2017-03-02" "2017-03-03" "2017-03-06" "2017-03-07" "2017-03-08"
#> [46] "2017-03-09" "2017-03-10" "2017-03-13" "2017-03-14" "2017-03-15"
#> [ reached 'max' / getOption("max.print") -- omitted 453 entries ]
# Weekday Sequence + Removing Business Holidays
tk_make_weekday_sequence("2017", "2018", remove_holidays = TRUE)
#> [1] "2017-01-03" "2017-01-04" "2017-01-05" "2017-01-06" "2017-01-09"
#> [6] "2017-01-10" "2017-01-11" "2017-01-12" "2017-01-13" "2017-01-17"
#> [11] "2017-01-18" "2017-01-19" "2017-01-20" "2017-01-23" "2017-01-24"
#> [16] "2017-01-25" "2017-01-26" "2017-01-27" "2017-01-30" "2017-01-31"
#> [21] "2017-02-01" "2017-02-02" "2017-02-03" "2017-02-06" "2017-02-07"
#> [26] "2017-02-08" "2017-02-09" "2017-02-10" "2017-02-13" "2017-02-14"
#> [31] "2017-02-15" "2017-02-16" "2017-02-17" "2017-02-21" "2017-02-22"
#> [36] "2017-02-23" "2017-02-24" "2017-02-27" "2017-02-28" "2017-03-01"
#> [41] "2017-03-02" "2017-03-03" "2017-03-06" "2017-03-07" "2017-03-08"
#> [46] "2017-03-09" "2017-03-10" "2017-03-13" "2017-03-14" "2017-03-15"
#> [ reached 'max' / getOption("max.print") -- omitted 453 entries ]
# ---- COMBINE HOLIDAYS WITH MAKE FUTURE TIMESERIES FROM EXISTING ----
# - A common machine learning application is creating a future time series data set
# from an existing
# Create index of days that FB stock will be traded in 2017 based on 2016 + holidays
FB_tbl <- FANG %>% dplyr::filter(symbol == "FB")
holidays <- tk_make_holiday_sequence(
start_date = "2016",
end_date = "2017",
calendar = "NYSE")
weekends <- tk_make_weekend_sequence(
start_date = "2016",
end_date = "2017")
# Remove holidays and weekends with skip_values
# We could also remove weekends with inspect_weekdays = TRUE
FB_tbl %>%
tk_index() %>%
tk_make_future_timeseries(length_out = 366,
skip_values = c(holidays, weekends))
#> The following `skip_values` were not in the future date sequence: 2016-01-01, 2016-01-18, 2016-02-15, 2016-03-25, 2016-05-30, 2016-07-04, 2016-09-05, 2016-11-24, 2016-12-26, 2016-01-02, 2016-01-03, 2016-01-09, 2016-01-10, 2016-01-16, 2016-01-17, 2016-01-23, 2016-01-24, 2016-01-30, 2016-01-31, 2016-02-06, 2016-02-07, 2016-02-13, 2016-02-14, 2016-02-20, 2016-02-21, 2016-02-27, 2016-02-28, 2016-03-05, 2016-03-06, 2016-03-12, 2016-03-13, 2016-03-19, 2016-03-20, 2016-03-26, 2016-03-27, 2016-04-02, 2016-04-03, 2016-04-09, 2016-04-10, 2016-04-16, 2016-04-17, 2016-04-23, 2016-04-24, 2016-04-30, 2016-05-01, 2016-05-07, 2016-05-08, 2016-05-14, 2016-05-15, 2016-05-21, 2016-05-22, 2016-05-28, 2016-05-29, 2016-06-04, 2016-06-05, 2016-06-11, 2016-06-12, 2016-06-18, 2016-06-19, 2016-06-25, 2016-06-26, 2016-07-02, 2016-07-03, 2016-07-09, 2016-07-10, 2016-07-16, 2016-07-17, 2016-07-23, 2016-07-24, 2016-07-30, 2016-07-31, 2016-08-06, 2016-08-07, 2016-08-13, 2016-08-14, 2016-08-20, 2016-08-21, 2016-08-27, 2016-08-28, 2016-09-03, 2016-09-04, 2016-09-10, 2016-09-11, 2016-09-17, 2016-09-18, 2016-09-24, 2016-09-25, 2016-10-01, 2016-10-02, 2016-10-08, 2016-10-09, 2016-10-15, 2016-10-16, 2016-10-22, 2016-10-23, 2016-10-29, 2016-10-30, 2016-11-05, 2016-11-06, 2016-11-12, 2016-11-13, 2016-11-19, 2016-11-20, 2016-11-26, 2016-11-27, 2016-12-03, 2016-12-04, 2016-12-10, 2016-12-11, 2016-12-17, 2016-12-18, 2016-12-24, 2016-12-25
#> [1] "2017-01-03" "2017-01-04" "2017-01-05" "2017-01-06" "2017-01-09"
#> [6] "2017-01-10" "2017-01-11" "2017-01-12" "2017-01-13" "2017-01-17"
#> [11] "2017-01-18" "2017-01-19" "2017-01-20" "2017-01-23" "2017-01-24"
#> [16] "2017-01-25" "2017-01-26" "2017-01-27" "2017-01-30" "2017-01-31"
#> [21] "2017-02-01" "2017-02-02" "2017-02-03" "2017-02-06" "2017-02-07"
#> [26] "2017-02-08" "2017-02-09" "2017-02-10" "2017-02-13" "2017-02-14"
#> [31] "2017-02-15" "2017-02-16" "2017-02-17" "2017-02-21" "2017-02-22"
#> [36] "2017-02-23" "2017-02-24" "2017-02-27" "2017-02-28" "2017-03-01"
#> [41] "2017-03-02" "2017-03-03" "2017-03-06" "2017-03-07" "2017-03-08"
#> [46] "2017-03-09" "2017-03-10" "2017-03-13" "2017-03-14" "2017-03-15"
#> [ reached 'max' / getOption("max.print") -- omitted 316 entries ]
options(max.print = options_old)