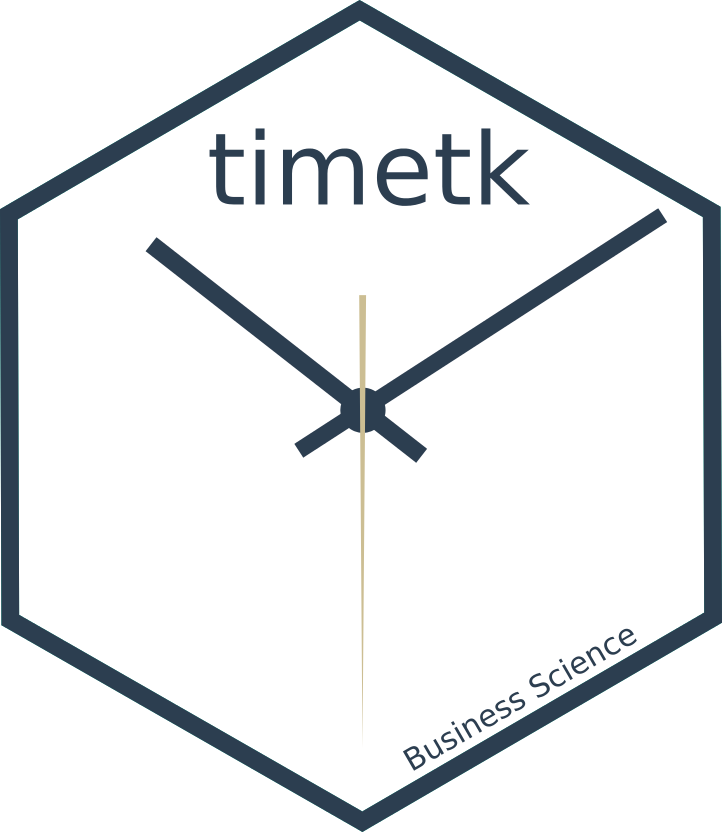
Make future time series from existing
Source:R/make-tk_make_timeseries_future.R
tk_make_future_timeseries.Rd
Make future time series from existing
Usage
tk_make_future_timeseries(
idx,
length_out,
inspect_weekdays = FALSE,
inspect_months = FALSE,
skip_values = NULL,
insert_values = NULL,
n_future = NULL
)
Arguments
- idx
A vector of dates
- length_out
Number of future observations. Can be numeric number or a phrase like "1 year".
- inspect_weekdays
Uses a logistic regression algorithm to inspect whether certain weekdays (e.g. weekends) should be excluded from the future dates. Default is
FALSE
.- inspect_months
Uses a logistic regression algorithm to inspect whether certain days of months (e.g. last two weeks of year or seasonal days) should be excluded from the future dates. Default is
FALSE
.- skip_values
A vector of same class as
idx
of timeseries values to skip.- insert_values
A vector of same class as
idx
of timeseries values to insert.- n_future
(DEPRECATED) Number of future observations. Can be numeric number or a phrase like "1 year".
Details
Future Sequences
tk_make_future_timeseries
returns a time series based
on the input index frequency and attributes.
Specifying Length of Future Observations
The argument length_out
determines how many future index observations to compute. It can be specified
as:
A numeric value - the number of future observations to return.
The number of observations returned is always equal to the value the user inputs.
The end date can vary based on the number of timestamps chosen.
A time-based phrase - The duration into the future to include (e.g. "6 months" or "30 minutes").
The duration defines the end date for observations.
The end date will not change and those timestamps that fall within the end date will be returned (e.g. a quarterly time series will return 4 quarters if
length_out = "1 year"
).The number of observations will vary to fit within the end date.
Weekday and Month Inspection
The inspect_weekdays
and inspect_months
arguments apply to "daily" (scale = "day") data
(refer to tk_get_timeseries_summary()
to get the index scale).
The
inspect_weekdays
argument is useful in determining missing days of the week that occur on a weekly frequency such as every week, every other week, and so on. It's recommended to have at least 60 days to use this option.The
inspect_months
argument is useful in determining missing days of the month, quarter or year; however, the algorithm can inadvertently select incorrect dates if the pattern is erratic.
Skipping / Inserting Values
The skip_values
and insert_values
arguments can be used to remove and add
values into the series of future times. The values must be the same format as the idx
class.
The
skip_values
argument useful for passing holidays or special index values that should be excluded from the future time series.The
insert_values
argument is useful for adding values back that the algorithm may have excluded.
See also
Making Time Series:
tk_make_timeseries()
Working with Holidays & Weekends:
tk_make_holiday_sequence()
,tk_make_weekend_sequence()
,tk_make_weekday_sequence()
Working with Timestamp Index:
tk_index()
,tk_get_timeseries_summary()
,tk_get_timeseries_signature()
Examples
library(dplyr)
# Basic example - By 3 seconds
idx <- tk_make_timeseries("2016-01-01 00:00:00", by = "3 sec", length_out = 3)
idx
#> [1] "2016-01-01 00:00:00 UTC" "2016-01-01 00:00:03 UTC"
#> [3] "2016-01-01 00:00:06 UTC"
# Make next three timestamps in series
idx %>% tk_make_future_timeseries(length_out = 3)
#> [1] "2016-01-01 00:00:09 UTC" "2016-01-01 00:00:12 UTC"
#> [3] "2016-01-01 00:00:15 UTC"
# Make next 6 seconds of timestamps from the next timestamp
idx %>% tk_make_future_timeseries(length_out = "6 sec")
#> [1] "2016-01-01 00:00:09 UTC" "2016-01-01 00:00:12 UTC"
# Basic Example - By 1 Month
idx <- tk_make_timeseries("2016-01-01", by = "1 month",
length_out = "12 months")
idx
#> [1] "2016-01-01" "2016-02-01" "2016-03-01" "2016-04-01" "2016-05-01"
#> [6] "2016-06-01" "2016-07-01" "2016-08-01" "2016-09-01" "2016-10-01"
#> [11] "2016-11-01" "2016-12-01" "2017-01-01"
# Make 12 months of timestamps from the next timestamp
idx %>% tk_make_future_timeseries(length_out = "12 months")
#> [1] "2017-02-01" "2017-03-01" "2017-04-01" "2017-05-01" "2017-06-01"
#> [6] "2017-07-01" "2017-08-01" "2017-09-01" "2017-10-01" "2017-11-01"
#> [11] "2017-12-01" "2018-01-01"
# --- APPLICATION ---
# - Combine holiday sequences with future sequences
# Create index of days that FB stock will be traded in 2017 based on 2016 + holidays
FB_tbl <- FANG %>% dplyr::filter(symbol == "FB")
holidays <- tk_make_holiday_sequence(
start_date = "2017-01-01",
end_date = "2017-12-31",
calendar = "NYSE")
# Remove holidays with skip_values, and remove weekends with inspect_weekdays = TRUE
FB_tbl %>%
tk_index() %>%
tk_make_future_timeseries(length_out = "1 year",
inspect_weekdays = TRUE,
skip_values = holidays)
#> [1] "2017-01-03" "2017-01-04" "2017-01-05" "2017-01-06" "2017-01-09"
#> [6] "2017-01-10" "2017-01-11" "2017-01-12" "2017-01-13" "2017-01-17"
#> [11] "2017-01-18" "2017-01-19" "2017-01-20" "2017-01-23" "2017-01-24"
#> [16] "2017-01-25" "2017-01-26" "2017-01-27" "2017-01-30" "2017-01-31"
#> [21] "2017-02-01" "2017-02-02" "2017-02-03" "2017-02-06" "2017-02-07"
#> [26] "2017-02-08" "2017-02-09" "2017-02-10" "2017-02-13" "2017-02-14"
#> [31] "2017-02-15" "2017-02-16" "2017-02-17" "2017-02-21" "2017-02-22"
#> [36] "2017-02-23" "2017-02-24" "2017-02-27" "2017-02-28" "2017-03-01"
#> [41] "2017-03-02" "2017-03-03" "2017-03-06" "2017-03-07" "2017-03-08"
#> [46] "2017-03-09" "2017-03-10" "2017-03-13" "2017-03-14" "2017-03-15"
#> [51] "2017-03-16" "2017-03-17" "2017-03-20" "2017-03-21" "2017-03-22"
#> [56] "2017-03-23" "2017-03-24" "2017-03-27" "2017-03-28" "2017-03-29"
#> [61] "2017-03-30" "2017-03-31" "2017-04-03" "2017-04-04" "2017-04-05"
#> [66] "2017-04-06" "2017-04-07" "2017-04-10" "2017-04-11" "2017-04-12"
#> [71] "2017-04-13" "2017-04-17" "2017-04-18" "2017-04-19" "2017-04-20"
#> [76] "2017-04-21" "2017-04-24" "2017-04-25" "2017-04-26" "2017-04-27"
#> [81] "2017-04-28" "2017-05-01" "2017-05-02" "2017-05-03" "2017-05-04"
#> [86] "2017-05-05" "2017-05-08" "2017-05-09" "2017-05-10" "2017-05-11"
#> [91] "2017-05-12" "2017-05-15" "2017-05-16" "2017-05-17" "2017-05-18"
#> [96] "2017-05-19" "2017-05-22" "2017-05-23" "2017-05-24" "2017-05-25"
#> [101] "2017-05-26" "2017-05-30" "2017-05-31" "2017-06-01" "2017-06-02"
#> [106] "2017-06-05" "2017-06-06" "2017-06-07" "2017-06-08" "2017-06-09"
#> [111] "2017-06-12" "2017-06-13" "2017-06-14" "2017-06-15" "2017-06-16"
#> [116] "2017-06-19" "2017-06-20" "2017-06-21" "2017-06-22" "2017-06-23"
#> [121] "2017-06-26" "2017-06-27" "2017-06-28" "2017-06-29" "2017-06-30"
#> [126] "2017-07-03" "2017-07-05" "2017-07-06" "2017-07-07" "2017-07-10"
#> [131] "2017-07-11" "2017-07-12" "2017-07-13" "2017-07-14" "2017-07-17"
#> [136] "2017-07-18" "2017-07-19" "2017-07-20" "2017-07-21" "2017-07-24"
#> [141] "2017-07-25" "2017-07-26" "2017-07-27" "2017-07-28" "2017-07-31"
#> [146] "2017-08-01" "2017-08-02" "2017-08-03" "2017-08-04" "2017-08-07"
#> [151] "2017-08-08" "2017-08-09" "2017-08-10" "2017-08-11" "2017-08-14"
#> [156] "2017-08-15" "2017-08-16" "2017-08-17" "2017-08-18" "2017-08-21"
#> [161] "2017-08-22" "2017-08-23" "2017-08-24" "2017-08-25" "2017-08-28"
#> [166] "2017-08-29" "2017-08-30" "2017-08-31" "2017-09-01" "2017-09-05"
#> [171] "2017-09-06" "2017-09-07" "2017-09-08" "2017-09-11" "2017-09-12"
#> [176] "2017-09-13" "2017-09-14" "2017-09-15" "2017-09-18" "2017-09-19"
#> [181] "2017-09-20" "2017-09-21" "2017-09-22" "2017-09-25" "2017-09-26"
#> [186] "2017-09-27" "2017-09-28" "2017-09-29" "2017-10-02" "2017-10-03"
#> [191] "2017-10-04" "2017-10-05" "2017-10-06" "2017-10-09" "2017-10-10"
#> [196] "2017-10-11" "2017-10-12" "2017-10-13" "2017-10-16" "2017-10-17"
#> [201] "2017-10-18" "2017-10-19" "2017-10-20" "2017-10-23" "2017-10-24"
#> [206] "2017-10-25" "2017-10-26" "2017-10-27" "2017-10-30" "2017-10-31"
#> [211] "2017-11-01" "2017-11-02" "2017-11-03" "2017-11-06" "2017-11-07"
#> [216] "2017-11-08" "2017-11-09" "2017-11-10" "2017-11-13" "2017-11-14"
#> [221] "2017-11-15" "2017-11-16" "2017-11-17" "2017-11-20" "2017-11-21"
#> [226] "2017-11-22" "2017-11-24" "2017-11-27" "2017-11-28" "2017-11-29"
#> [231] "2017-11-30" "2017-12-01" "2017-12-04" "2017-12-05" "2017-12-06"
#> [236] "2017-12-07" "2017-12-08" "2017-12-11" "2017-12-12" "2017-12-13"
#> [241] "2017-12-14" "2017-12-15" "2017-12-18" "2017-12-19" "2017-12-20"
#> [246] "2017-12-21" "2017-12-22" "2017-12-26" "2017-12-27" "2017-12-28"
#> [251] "2017-12-29"