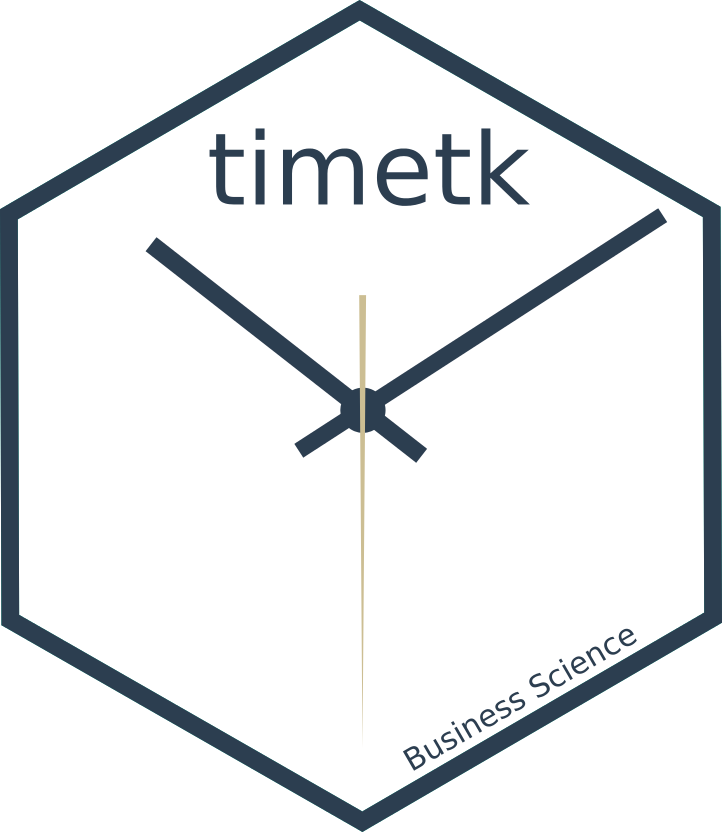
Add many rolling window calculations to the data
Source:R/augment-tk_augment_slidify.R
tk_augment_slidify.Rd
Quickly use any function as a rolling function and apply to multiple .periods
.
Works with dplyr
groups too.
Usage
tk_augment_slidify(
.data,
.value,
.period,
.f,
...,
.align = c("center", "left", "right"),
.partial = FALSE,
.names = "auto"
)
Arguments
- .data
A tibble.
- .value
One or more column(s) to have a transformation applied. Usage of
tidyselect
functions (e.g.contains()
) can be used to select multiple columns.- .period
One or more periods for the rolling window(s)
- .f
A summary
[function / formula]
,- ...
Optional arguments for the summary function
- .align
Rolling functions generate
.period - 1
fewer values than the incoming vector. Thus, the vector needs to be aligned. Select one of "center", "left", or "right".- .partial
.partial Should the moving window be allowed to return partial (incomplete) windows instead of
NA
values. Set to FALSE by default, but can be switched to TRUE to removeNA
's.- .names
A vector of names for the new columns. Must be of same length as
.period
. Default is "auto".
Details
tk_augment_slidify()
scales the slidify_vec()
function to multiple
time series .periods
. See slidify_vec()
for examples and usage of the core function
arguments.
See also
Augment Operations:
tk_augment_timeseries_signature()
- Group-wise augmentation of timestamp featurestk_augment_holiday_signature()
- Group-wise augmentation of holiday featurestk_augment_slidify()
- Group-wise augmentation of rolling functionstk_augment_lags()
- Group-wise augmentation of lagged datatk_augment_differences()
- Group-wise augmentation of differenced datatk_augment_fourier()
- Group-wise augmentation of fourier series
Underlying Function:
slidify_vec()
- The underlying function that powerstk_augment_slidify()
Examples
library(dplyr)
# Single Column | Multiple Rolling Windows
FANG %>%
select(symbol, date, adjusted) %>%
group_by(symbol) %>%
tk_augment_slidify(
.value = contains("adjust"),
# Multiple rolling windows
.period = c(10, 30, 60, 90),
.f = mean,
.partial = TRUE,
.names = stringr::str_c("MA_", c(10, 30, 60, 90))
) %>%
ungroup()
#> # A tibble: 4,032 × 7
#> symbol date adjusted MA_10 MA_30 MA_60 MA_90
#> <chr> <date> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 FB 2013-01-02 28 28.9 30.0 29.7 29.1
#> 2 FB 2013-01-03 27.8 29.3 30.1 29.7 29.1
#> 3 FB 2013-01-04 28.8 29.6 30.2 29.7 29.0
#> 4 FB 2013-01-07 29.4 29.7 30.2 29.6 29.0
#> 5 FB 2013-01-08 29.1 29.8 30.3 29.6 29.0
#> 6 FB 2013-01-09 30.6 30.0 30.3 29.5 28.9
#> 7 FB 2013-01-10 31.3 30.2 30.3 29.4 28.9
#> 8 FB 2013-01-11 31.7 30.3 30.2 29.4 28.8
#> 9 FB 2013-01-14 31.0 30.4 30.1 29.3 28.8
#> 10 FB 2013-01-15 30.1 30.6 30.1 29.3 28.7
#> # ℹ 4,022 more rows
# Multiple Columns | Multiple Rolling Windows
FANG %>%
select(symbol, date, adjusted, volume) %>%
group_by(symbol) %>%
tk_augment_slidify(
.value = c(adjusted, volume),
.period = c(10, 30, 60, 90),
.f = mean,
.partial = TRUE
) %>%
ungroup()
#> # A tibble: 4,032 × 12
#> symbol date adjusted volume adjusted_roll_10 volume_roll_10
#> <chr> <date> <dbl> <dbl> <dbl> <dbl>
#> 1 FB 2013-01-02 28 69846400 28.9 73357200
#> 2 FB 2013-01-03 27.8 63140600 29.3 76494229.
#> 3 FB 2013-01-04 28.8 72715400 29.6 78132200
#> 4 FB 2013-01-07 29.4 83781800 29.7 80438933.
#> 5 FB 2013-01-08 29.1 45871300 29.8 89719300
#> 6 FB 2013-01-09 30.6 104787700 30.0 90267930
#> 7 FB 2013-01-10 31.3 95316400 30.2 87979540
#> 8 FB 2013-01-11 31.7 89598000 30.3 85671150
#> 9 FB 2013-01-14 31.0 98892800 30.4 82817300
#> 10 FB 2013-01-15 30.1 173242600 30.6 83120150
#> # ℹ 4,022 more rows
#> # ℹ 6 more variables: adjusted_roll_30 <dbl>, volume_roll_30 <dbl>,
#> # adjusted_roll_60 <dbl>, volume_roll_60 <dbl>, adjusted_roll_90 <dbl>,
#> # volume_roll_90 <dbl>