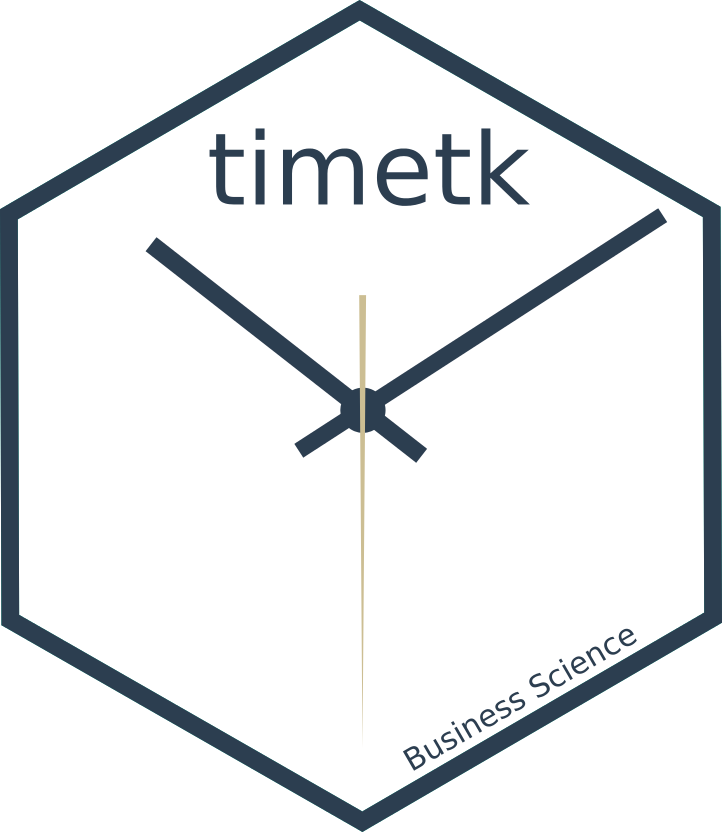
Between (For Time Series): Range detection for date or date-time sequences
Source:R/dplyr-between_time.R
between_time.Rd
The easiest way to filter time series date or date-time vectors. Returns a
logical vector indicating which date or date-time values are within a range.
See filter_by_time()
for the data.frame
(tibble
) implementation.
Value
A logical
vector the same length as index
indicating whether or not
the timestamp value was within the start_date
and end_date
range.
Details
Pure Time Series Filtering Flexibilty
The start_date
and end_date
parameters are designed with flexibility in mind.
Each side of the time_formula
is specified as the character
'YYYY-MM-DD HH:MM:SS'
, but powerful shorthand is available.
Some examples are:
Year:
start_date = '2013', end_date = '2015'
Month:
start_date = '2013-01', end_date = '2016-06'
Day:
start_date = '2013-01-05', end_date = '2016-06-04'
Second:
start_date = '2013-01-05 10:22:15', end_date = '2018-06-03 12:14:22'
Variations:
start_date = '2013', end_date = '2016-06'
Key Words: "start" and "end"
Use the keywords "start" and "end" as shorthand, instead of specifying the actual start and end values. Here are some examples:
Start of the series to end of 2015:
start_date = 'start', end_date = '2015'
Start of 2014 to end of series:
start_date = '2014', end_date = 'end'
Internal Calculations
All shorthand dates are expanded:
The
start_date
is expanded to be the first date in that periodThe
end_date
side is expanded to be the last date in that period
This means that the following examples are equivalent (assuming your index is a POSIXct):
start_date = '2015'
is equivalent tostart_date = '2015-01-01 + 00:00:00'
end_date = '2016'
is equivalent to2016-12-31 + 23:59:59'
References
This function is based on the
tibbletime::filter_time()
function developed by Davis Vaughan.
See also
Time-Based dplyr functions:
summarise_by_time()
- Easily summarise using a date column.mutate_by_time()
- Simplifies applying mutations by time windows.pad_by_time()
- Insert time series rows with regularly spaced timestampsfilter_by_time()
- Quickly filter using date ranges.filter_period()
- Apply filtering expressions inside periods (windows)slice_period()
- Apply slice inside periods (windows)condense_period()
- Convert to a different periodicitybetween_time()
- Range detection for date or date-time sequences.slidify()
- Turn any function into a sliding (rolling) function
Examples
library(dplyr)
index_daily <- tk_make_timeseries("2016-01-01", "2017-01-01", by = "day")
index_min <- tk_make_timeseries("2016-01-01", "2017-01-01", by = "min")
# How it works
# - Returns TRUE/FALSE length of index
# - Use sum() to tally the number of TRUE values
index_daily %>% between_time("start", "2016-01") %>% sum()
#> [1] 31
# ---- INDEX SLICING ----
# Daily Series: Month of January 2016
index_daily[index_daily %>% between_time("start", "2016-01")]
#> [1] "2016-01-01" "2016-01-02" "2016-01-03" "2016-01-04" "2016-01-05"
#> [6] "2016-01-06" "2016-01-07" "2016-01-08" "2016-01-09" "2016-01-10"
#> [11] "2016-01-11" "2016-01-12" "2016-01-13" "2016-01-14" "2016-01-15"
#> [16] "2016-01-16" "2016-01-17" "2016-01-18" "2016-01-19" "2016-01-20"
#> [21] "2016-01-21" "2016-01-22" "2016-01-23" "2016-01-24" "2016-01-25"
#> [26] "2016-01-26" "2016-01-27" "2016-01-28" "2016-01-29" "2016-01-30"
#> [31] "2016-01-31"
# Daily Series: March 1st - June 15th, 2016
index_daily[index_daily %>% between_time("2016-03", "2016-06-15")]
#> [1] "2016-03-01" "2016-03-02" "2016-03-03" "2016-03-04" "2016-03-05"
#> [6] "2016-03-06" "2016-03-07" "2016-03-08" "2016-03-09" "2016-03-10"
#> [11] "2016-03-11" "2016-03-12" "2016-03-13" "2016-03-14" "2016-03-15"
#> [16] "2016-03-16" "2016-03-17" "2016-03-18" "2016-03-19" "2016-03-20"
#> [21] "2016-03-21" "2016-03-22" "2016-03-23" "2016-03-24" "2016-03-25"
#> [26] "2016-03-26" "2016-03-27" "2016-03-28" "2016-03-29" "2016-03-30"
#> [31] "2016-03-31" "2016-04-01" "2016-04-02" "2016-04-03" "2016-04-04"
#> [36] "2016-04-05" "2016-04-06" "2016-04-07" "2016-04-08" "2016-04-09"
#> [41] "2016-04-10" "2016-04-11" "2016-04-12" "2016-04-13" "2016-04-14"
#> [46] "2016-04-15" "2016-04-16" "2016-04-17" "2016-04-18" "2016-04-19"
#> [51] "2016-04-20" "2016-04-21" "2016-04-22" "2016-04-23" "2016-04-24"
#> [56] "2016-04-25" "2016-04-26" "2016-04-27" "2016-04-28" "2016-04-29"
#> [61] "2016-04-30" "2016-05-01" "2016-05-02" "2016-05-03" "2016-05-04"
#> [66] "2016-05-05" "2016-05-06" "2016-05-07" "2016-05-08" "2016-05-09"
#> [71] "2016-05-10" "2016-05-11" "2016-05-12" "2016-05-13" "2016-05-14"
#> [76] "2016-05-15" "2016-05-16" "2016-05-17" "2016-05-18" "2016-05-19"
#> [81] "2016-05-20" "2016-05-21" "2016-05-22" "2016-05-23" "2016-05-24"
#> [86] "2016-05-25" "2016-05-26" "2016-05-27" "2016-05-28" "2016-05-29"
#> [91] "2016-05-30" "2016-05-31" "2016-06-01" "2016-06-02" "2016-06-03"
#> [96] "2016-06-04" "2016-06-05" "2016-06-06" "2016-06-07" "2016-06-08"
#> [101] "2016-06-09" "2016-06-10" "2016-06-11" "2016-06-12" "2016-06-13"
#> [106] "2016-06-14" "2016-06-15"
# Minute Series:
index_min[index_min %>% between_time("2016-02-01 12:00", "2016-02-01 13:00")]
#> [1] "2016-02-01 12:00:00 UTC" "2016-02-01 12:01:00 UTC"
#> [3] "2016-02-01 12:02:00 UTC" "2016-02-01 12:03:00 UTC"
#> [5] "2016-02-01 12:04:00 UTC" "2016-02-01 12:05:00 UTC"
#> [7] "2016-02-01 12:06:00 UTC" "2016-02-01 12:07:00 UTC"
#> [9] "2016-02-01 12:08:00 UTC" "2016-02-01 12:09:00 UTC"
#> [11] "2016-02-01 12:10:00 UTC" "2016-02-01 12:11:00 UTC"
#> [13] "2016-02-01 12:12:00 UTC" "2016-02-01 12:13:00 UTC"
#> [15] "2016-02-01 12:14:00 UTC" "2016-02-01 12:15:00 UTC"
#> [17] "2016-02-01 12:16:00 UTC" "2016-02-01 12:17:00 UTC"
#> [19] "2016-02-01 12:18:00 UTC" "2016-02-01 12:19:00 UTC"
#> [21] "2016-02-01 12:20:00 UTC" "2016-02-01 12:21:00 UTC"
#> [23] "2016-02-01 12:22:00 UTC" "2016-02-01 12:23:00 UTC"
#> [25] "2016-02-01 12:24:00 UTC" "2016-02-01 12:25:00 UTC"
#> [27] "2016-02-01 12:26:00 UTC" "2016-02-01 12:27:00 UTC"
#> [29] "2016-02-01 12:28:00 UTC" "2016-02-01 12:29:00 UTC"
#> [31] "2016-02-01 12:30:00 UTC" "2016-02-01 12:31:00 UTC"
#> [33] "2016-02-01 12:32:00 UTC" "2016-02-01 12:33:00 UTC"
#> [35] "2016-02-01 12:34:00 UTC" "2016-02-01 12:35:00 UTC"
#> [37] "2016-02-01 12:36:00 UTC" "2016-02-01 12:37:00 UTC"
#> [39] "2016-02-01 12:38:00 UTC" "2016-02-01 12:39:00 UTC"
#> [41] "2016-02-01 12:40:00 UTC" "2016-02-01 12:41:00 UTC"
#> [43] "2016-02-01 12:42:00 UTC" "2016-02-01 12:43:00 UTC"
#> [45] "2016-02-01 12:44:00 UTC" "2016-02-01 12:45:00 UTC"
#> [47] "2016-02-01 12:46:00 UTC" "2016-02-01 12:47:00 UTC"
#> [49] "2016-02-01 12:48:00 UTC" "2016-02-01 12:49:00 UTC"
#> [51] "2016-02-01 12:50:00 UTC" "2016-02-01 12:51:00 UTC"
#> [53] "2016-02-01 12:52:00 UTC" "2016-02-01 12:53:00 UTC"
#> [55] "2016-02-01 12:54:00 UTC" "2016-02-01 12:55:00 UTC"
#> [57] "2016-02-01 12:56:00 UTC" "2016-02-01 12:57:00 UTC"
#> [59] "2016-02-01 12:58:00 UTC" "2016-02-01 12:59:00 UTC"
#> [61] "2016-02-01 13:00:00 UTC"
# ---- FILTERING WITH DPLYR ----
FANG %>%
group_by(symbol) %>%
filter(date %>% between_time("2016-01", "2016-01"))
#> # A tibble: 76 × 8
#> # Groups: symbol [4]
#> symbol date open high low close volume adjusted
#> <chr> <date> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 FB 2016-01-04 102. 102. 99.8 102. 37912400 102.
#> 2 FB 2016-01-05 103. 104. 102. 103. 23258200 103.
#> 3 FB 2016-01-06 101. 104. 101. 103. 25096200 103.
#> 4 FB 2016-01-07 100. 101. 97.3 97.9 45172900 97.9
#> 5 FB 2016-01-08 99.9 100. 97.0 97.3 35402300 97.3
#> 6 FB 2016-01-11 97.9 98.6 95.4 97.5 29932400 97.5
#> 7 FB 2016-01-12 99 100. 97.6 99.4 28395400 99.4
#> 8 FB 2016-01-13 101. 101. 95.2 95.4 33410600 95.4
#> 9 FB 2016-01-14 95.8 98.9 92.4 98.4 48658600 98.4
#> 10 FB 2016-01-15 94.0 96.4 93.5 95.0 45935600 95.0
#> # ℹ 66 more rows